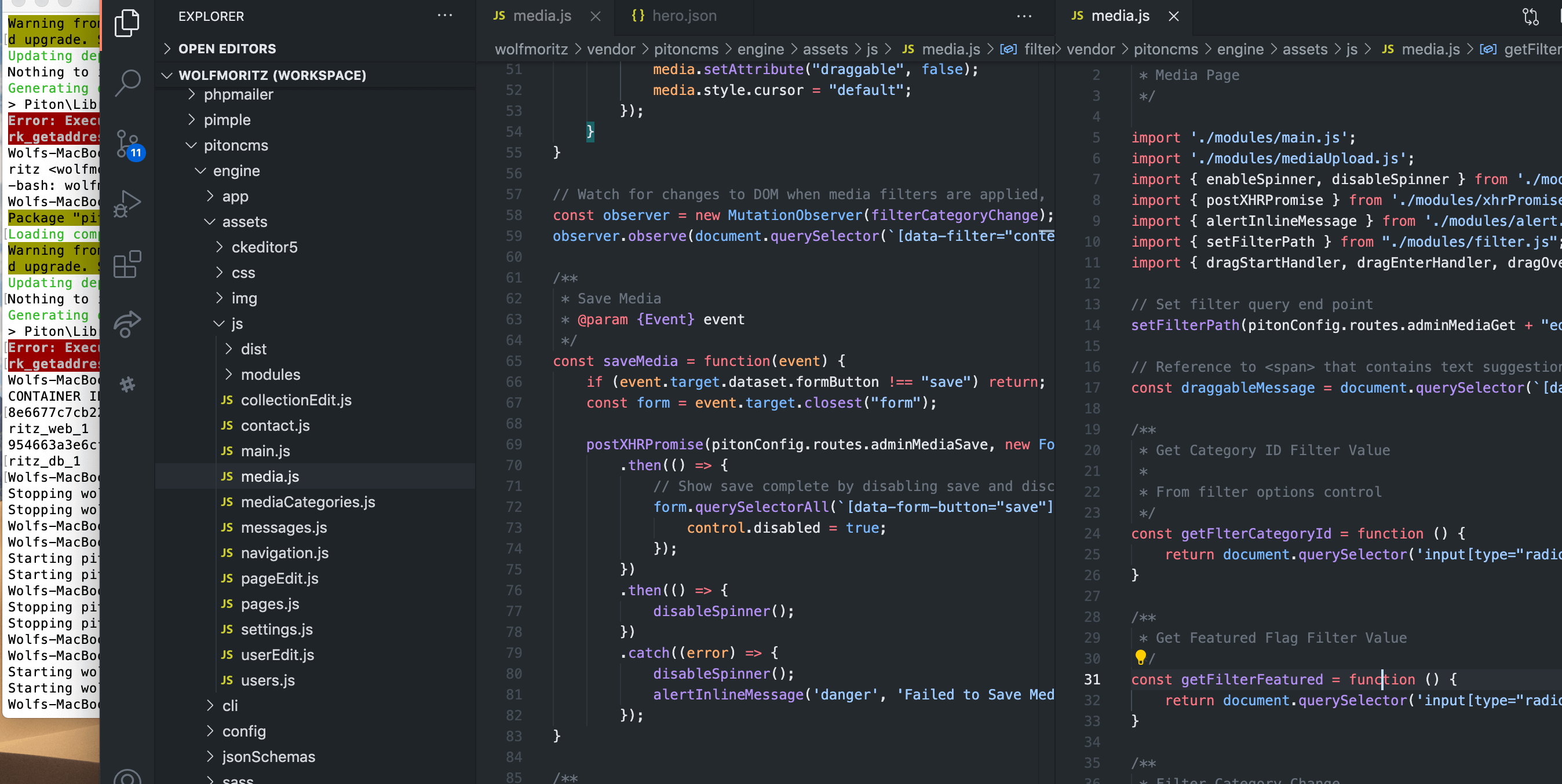
Lightweight PHP MySQL Session Handler
Note: This repo has been archived and is no longer supported. The package has been moved and updated for PHP7+ to PitonCMS/Session. I leave this here as an example.
Managing session state across a stateless protocol like HTTP has its challenges. PHP has a native session handler, and is easy to use, but I wanted something that persisted to the (MySQL) database. CodeIgniter has a great session class that uses a database table, but now most of my PHP development is more modular using Composer to manage dependencies, and I wanted something similar to CodeIgniter's session class.
So I assembled a modest PHP session handler class and made it available via Composer, or directly on GitHub. To get it by way of Composer, add this to your composer.json file and run composer install:
"require": {
"wolfmoritz/session": "~1.2.0"
}
The package requires a MySQL table to store session data (serialized to JSON) using the PDO driver for PHP. The session key is regenerated every 5 minutes, which is a configurable item.
When instantiated, the class will auto run making previously saved session data available, or allow you to save session data for future requests. The class also supports flash data, which is only available on the next request after which flash data is automatically removed (great for validation messages).
Basic methods include:
// Save simple key-value pair
$Session->setData('someKey', 'someValue');
// Save array of key-value pairs
$sessionData = array(
'feline' => 'cat',
'canine' => 'dog',
'lastModified' => 1422592486
);
$Session->setData($sessionData);
// Get one session item
$value = $Session->getData('someKey');
// Get all session items
$values = $Session->getData();
// Delete one session item
$Session->unsetData('someKey');
// Delete all session data
$Session->unsetData();
// Save simple key-value pair as flash data, or just the message
$Session->setFlashData('Something went wrong!');
$Session->setFlashData('alert', 'Something went very wrong!');
// Get one flash item
$value = $Session->getFlashData('alert');
// Get all flash items
$values = $Session->getFlashData();
You can read more about the session class on GitHub/wolfmoritz/session.